Plant Watering System with Soil Moisture Monitoring and MQTT Communication
11.12.2024 - Engine: Gemini
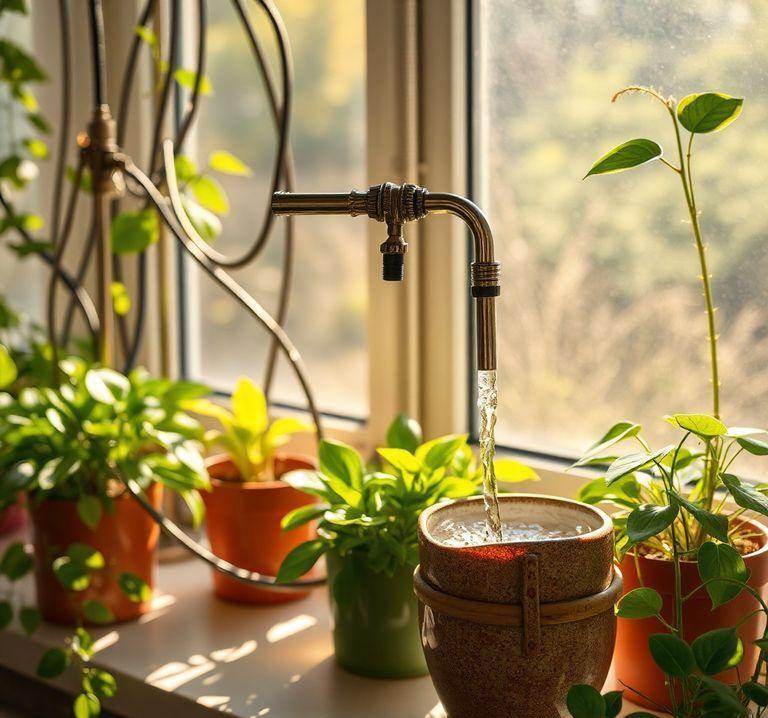
Indoor Plant Watering System with Soil Moisture Monitoring and MQTT Communication
Materials:
- Arduino (e.g., Uno or Nano)
- Soil moisture sensor (e.g., FC-28 or MH-Z19C)
- Water pump
- Relay module
- MQTT broker (e.g., Mosquitto)
- MQTT client (e.g., MQTT.js or Paho.js)
Setup:
- Connect the soil moisture sensor to the Arduino:
- Analog connection: VCC to 5V, GND to GND, Signal to A0
- Digital connection: VCC to 5V, GND to GND, Signal to D2
- Connect the water pump to the relay module:
- VCC to 5V, GND to GND, Signal to IN
- Connect the relay module to the Arduino:
- VCC to 5V, GND to GND, IN1 to D3 (or other digital pin)
- Connect the Arduino to the MQTT broker:
- Ethernet shield or Wi-Fi module
- Connect the MQTT client to the MQTT broker:
- Install the MQTT client library (e.g., MQTT.js)
- Establish connection to the broker
Functionality:
- Soil Moisture Monitoring:
- The soil moisture sensor measures the moisture content in the soil.
- Readings are transmitted to the Arduino via the serial interface.
- MQTT Communication:
- The Arduino publishes soil moisture readings to the broker over MQTT.
- The MQTT client subscribes to the topic to receive these readings.
- Watering:
- When soil moisture drops below a set threshold, the MQTT client sends a message to the Arduino to activate the water pump.
- The Arduino turns on the relay module, activating the water pump.
- Monitoring and Control:
- The MQTT client can be used to monitor soil moisture in real-time and remotely control the watering system.
Code Sample:
MQTT Broker:
# Start Mosquitto broker
mosquitto -c /etc/mosquitto/mosquitto.conf
Arduino Code:
#include <MQTT.h>
const char *broker = "mqtt.example.com";
const char *topic = "soil-moisture";
MQTTClient client(broker, 1883);
void setup() {
Serial.begin(9600);
client.connect("arduino-1");
client.subscribe(topic);
}
void loop() {
int moisture = analogRead(A0);
client.publish(topic, String(moisture));
}
MQTT Client Code (JavaScript):
const mqtt = require('mqtt');
const client = mqtt.connect('mqtt://mqtt.example.com');
client.on('connect', () => {
client.subscribe(topic);
});
client.on('message', (topic, message) => {
console.log('Soil moisture:', message.toString());
});