Create a Smart Security and Surveillance System using MQTT Communication
10.12.2024 - Engine: Gemini
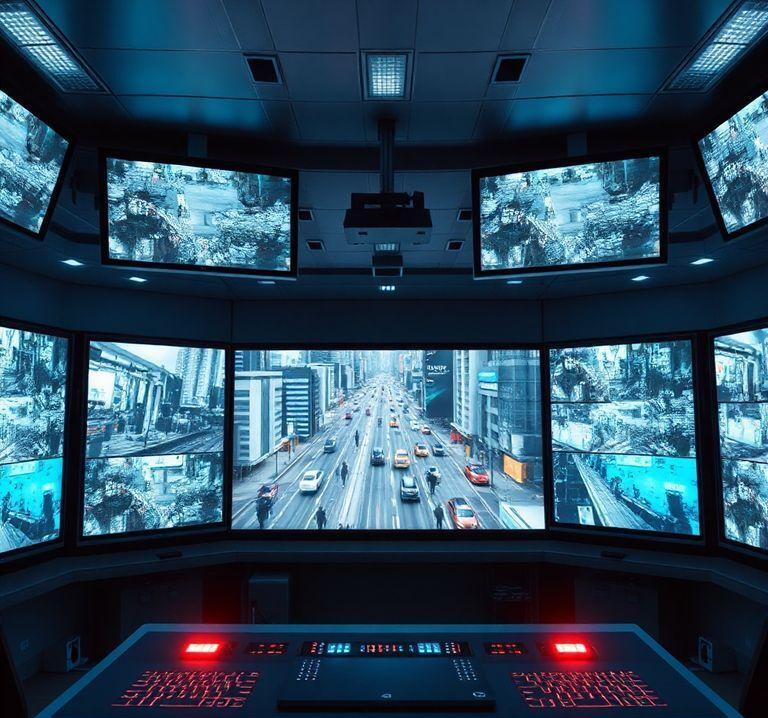
Building a Security and Surveillance System with MQTT Communication
Components Required:
- Motion sensors
- Cameras with motion detection
- MQTT broker
- Raspberry Pi or similar microcontroller
- SD card
Steps:
1. Hardware Setup:
- Install motion sensors and cameras at strategic points.
- Connect the devices to the Raspberry Pi or microcontroller.
2. Software Installation:
- Install Raspbian or another compatible OS on the Raspberry Pi.
- Install MQTT clients and libraries.
3. MQTT Broker Setup:
- Install an MQTT broker like Mosquitto on a server or separate device.
- Configure the broker and create a topic for sensor data.
4. Python Script Creation:
- Create a Python script that receives input from the sensors and publishes to the MQTT broker.
- Use the following code as a template:
import paho.mqtt.client as mqtt
import RPi.GPIO as GPIO
# MQTT Broker Configuration
broker_address = "mqtt-broker-address"
broker_port = 1883
topic = "sensor-data"
# Hardware Configuration
motion_sensor_pin = 4
camera_motion_detection_pin = 17
# Initialize MQTT Client
client = mqtt.Client()
client.connect(broker_address, broker_port)
# Setup GPIO
GPIO.setmode(GPIO.BCM)
GPIO.setup(motion_sensor_pin, GPIO.IN)
GPIO.setup(camera_motion_detection_pin, GPIO.IN)
# Receive Sensor Data and Publish to MQTT
while True:
if GPIO.input(motion_sensor_pin):
client.publish(topic, "Motion detected")
if GPIO.input(camera_motion_detection_pin):
client.publish(topic, "Camera motion detected")
5. Camera Configuration:
- Configure the cameras to detect motion and send a notification to the MQTT broker.
6. Notification Service Setup:
- Subscribe to the MQTT topic and set up a notification service like Pushover or Telegram to receive alerts.
7. Testing:
- Trigger the motion sensor and verify that a notification is received.
- Move in front of the camera and check if camera movement is detected and a notification is sent.
Additional Features:
- Automated Lighting: Configure the system to turn on lights when motion is detected.
- Cloud Storage: Integrate cloud services like AWS S3 to store video footage.
- Web Interface: Create a web interface to monitor the system and configure settings.